Functions in Swift
Oct 18, 2024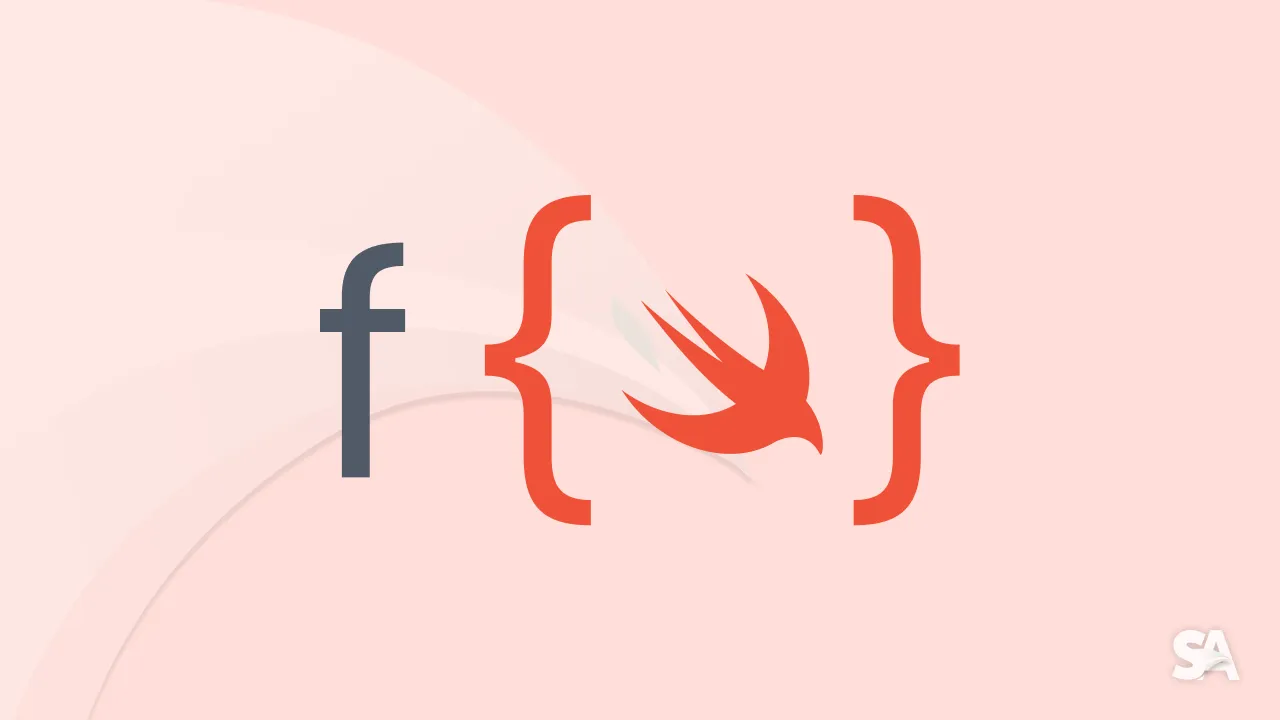
You can think of functions as a recipe. Just like how once you’ve written down the steps, you don’t have to figure out how to cook the dish again, you just follow the recipe. Similarly, once defined, you can call the function whenever needed without re-writing the entire logic.
In Swift, functions are an independent block of code that are used to perform specific tasks. Functions makes the code more structured and reusable.
Table of Contents
- How to declare a function in Swift
- Calling a function in Swift
- Passing Parameters to Swift function
- Default Parameter Values
- How to Return Values from Swift function
- Function Argument Label in Swift
- Excluding Argument Labels
How to declare a function in Swift
To define a function you simply have to use the func
keyword followed by the name of the function and a pair of parenthesis. Then, define the function content within curly braces.
func myInfo() {
var age = 18
let name = "Michael"
var line = ("Hi, I am " + "\(name) \n") + ("I am " + "\(age)")
print(line)
}
Calling a function in Swift
To call a function in Swift, you have to write the function name suffixed with parenthesis and can use it wherever required.
myInfo()
Output:
Hi, I am Michael
I am 18
Passing Parameters to Swift function
You learned how to write a function and call it. But what if you want to call the same function with some other name & age? Don't worry you don't have to define the same function again and again.
In Swift, functions can take input values, called parameters, which allow you to pass data into the function. These parameters act as placeholders that are filled with actual values when the function is called.
Each parameter has a name and a type, separated by a colon and it needs to be passed within the parentheses after the function name.
func tesla(model: String, year: Int) {
print("Tesla" + " \(model)" + " was released in the year" + " \(year)")
}
tesla(model: "Roadster", year: 2018)
Output:
Tesla Roadster was released in the year 2018
In the above code, model
is a parameter of type String
and year
is another parameter of type Int
. When the function is called the value of model
is passed as "Roadster" and year
is given a value of 2018.
Note: Order of the arguments passed to the function should be same as what is defined in the function definition. Otherwise, the compiler will throw an error.
Default Parameter Values
Sometimes you would want to provide a default value to your parameters so that even if you forget to pass the argument, the code shouln't break and Swift will use the default value that you've specified.
Defining a default value is similar to how you assign a value to a variable at the time of declaration.
func placeOrder(food: String, instructions: String = "Leave at door") {
print("Order placed for \(food). Instructions: \(instructions).")
}
placeOrder(food: "Pizza")
Output:
Order placed for Pizza. Instructions: Leave at door.
In the above code, even if you didn't pass the argument for instructions
, it is automatically taken as "Leave at door".
However, you can still override the default value by passing a specific argument.
placeOrder(food: "Burger", instructions: "Ring the bell")
Output:
Order placed for Burger. Instructions: Ring the bell.
How to Return Values from Swift function
In Swift, functions can not only take values as arguments but can also return values. Return values are useful when you want to process some data and use the result somewhere else in your code.
To specify that a function returns a value, you have to use the -> symbol followed by the return type in the function declaration. You also have to use the return
keyword within the function to return a particular value. The function can only return the type of variable stated as the return type.
func calculateInterest(principal: Double, rate: Double, years: Int) -> Double {
let interest = principal * rate * Double(years) / 100
return interest
}
let interestAmount = calculateInterest(principal: 10000, rate: 5, years: 3)
print("Interest earned: $\(interestAmount)")
Output:
Interest earned: $1500.0
The above function returns a value of type Double
which is nothing but the calculated interest. If you mention the return value type and don't return value in the function, the compiler will throw an error.
Function Argument Label in Swift
To make the functions more readable and easy to understand, Swift functions can have argument labels. Argument labels provide additional information about the parameters and are used to clarify what each argument represents when calling the function.
By default, if you don't specify the argument label, the parameter name itself is taken as the argument label.
An argument label is specified before a parameter name, and it's used only when the function is called while the parameter name is what you'll use within the function body.
func bookFlight(for passenger: String, at time: String, from city: String) {
print("\(passenger) booked a flight from \(city) at \(time).")
}
bookFlight(for: "Drake", at: "10:00 AM", from: "New York")
Output:
Drake booked a flight from New York at 10:00 AM.
In the above example, for
, at
and from
are argument labels used while calling the functions while passenger
, time
and city
are parameters which are used inside the function.
Excluding Argument Labels
If you want to avoid using the argument label for a parameter, you can simply use a "_" (underscore) as the argument label. In such a case, you can directly pass the value as argument without associating it with a name.
func bookFlight(_ passenger: String, at time: String, from city: String) {
print("\(passenger) booked a flight from \(city) at \(time).")
}
bookFlight("Drake", at: "10:00 AM", from: "New York")
Output:
Drake booked a flight from New York at 10:00 AM.
Where to go next?
Congratulations, today you have completed understanding, implementing, and learning one of the crucial topics of programming and, of course, Swift. We would highly recommend you to read about more related articles like Enums in Swift and Optionals and Unwrapping in Swift.